okay, so I need some more help.
The issue I am having is not so much the actual functioning, it is just the way the instructor wants it.
The first picture is the instructions from the course page:
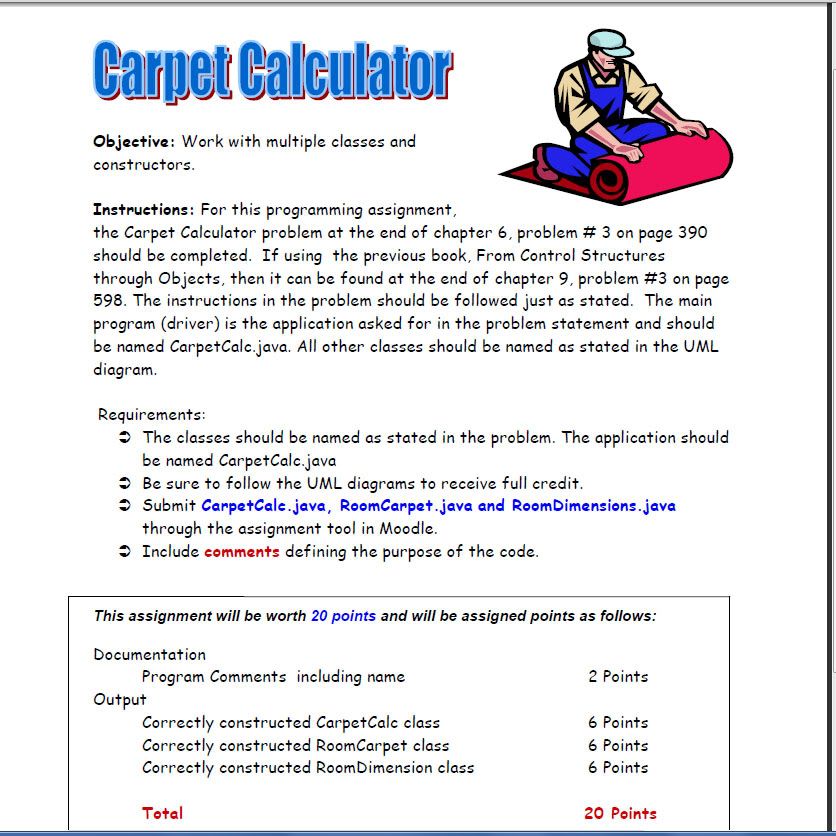
Now here is the text from the book:
The next picture is of the UML diagram:
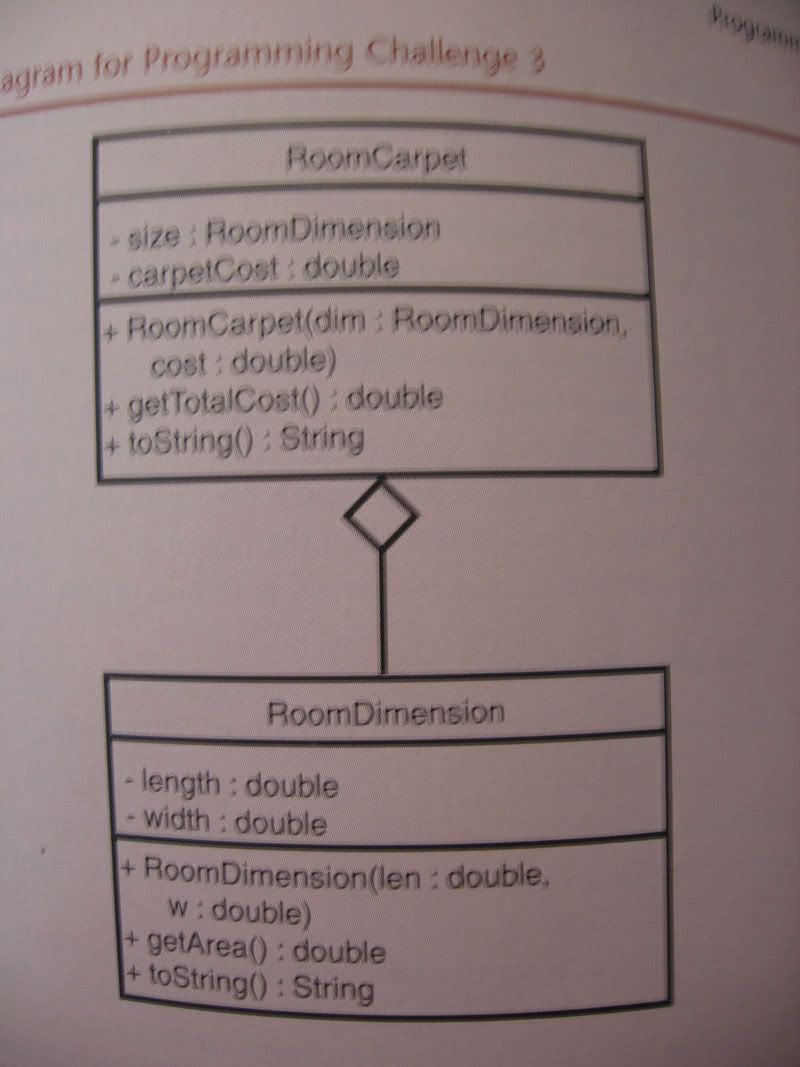
Now, I am having trouble following the UML diagram to the T. Because the way I read it, I should only have 3 methods in each class. My possible solution is to put additional methods inside the class.
The issue I am having is not so much the actual functioning, it is just the way the instructor wants it.
The first picture is the instructions from the course page:
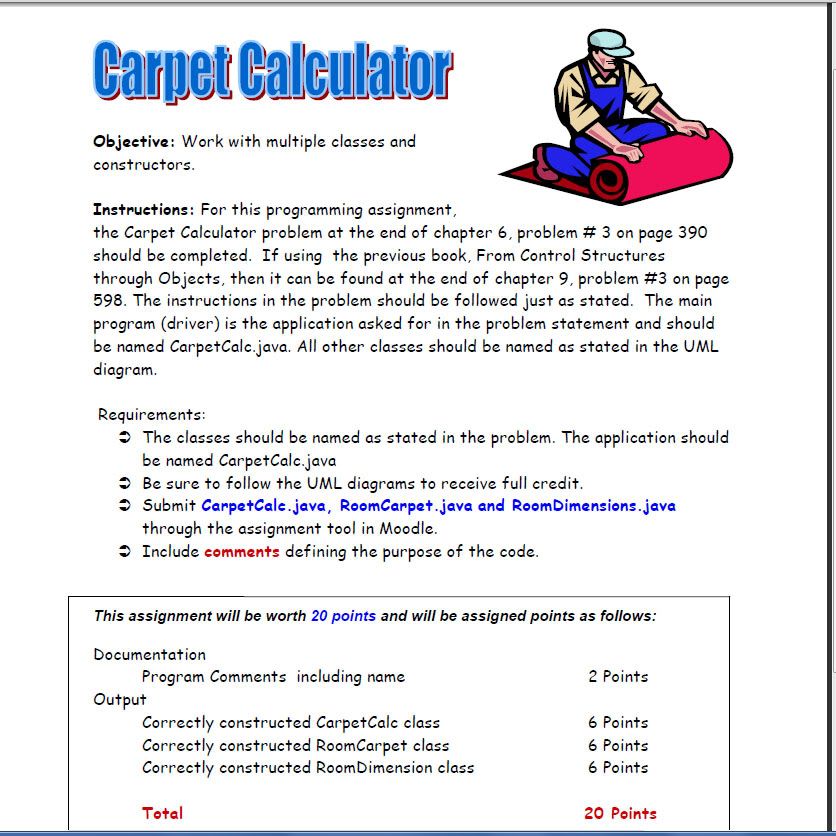
Now here is the text from the book:
Originally posted by Textbook
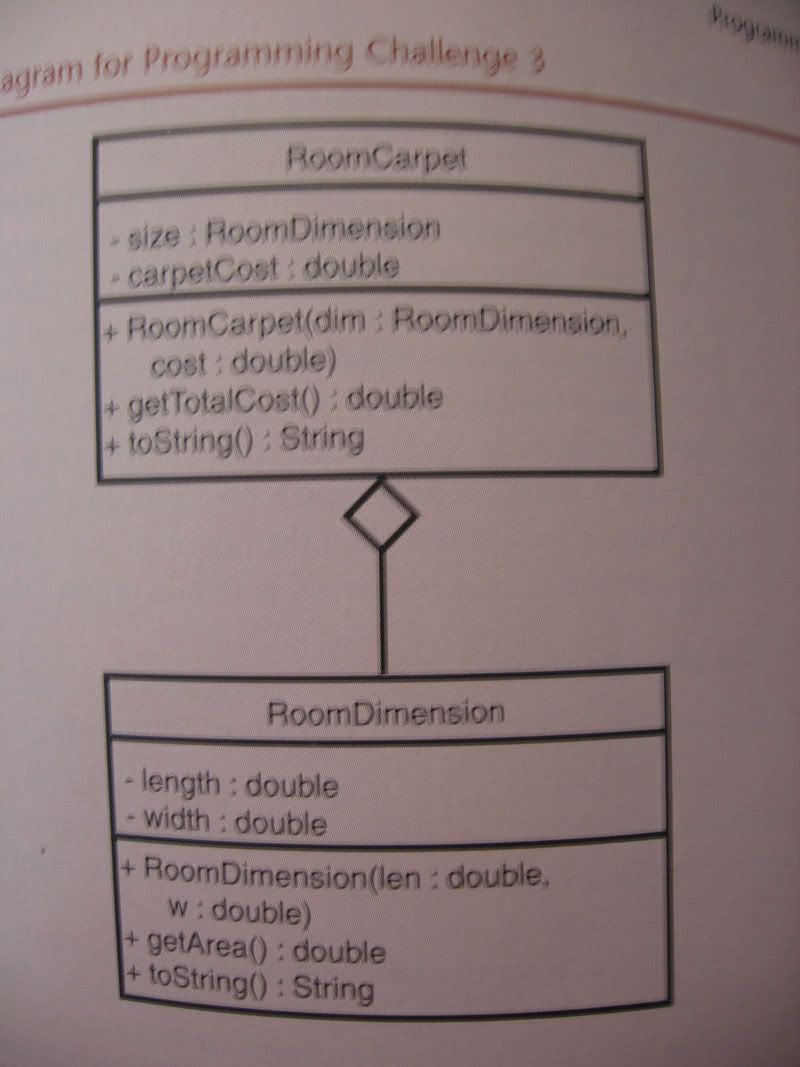
Now, I am having trouble following the UML diagram to the T. Because the way I read it, I should only have 3 methods in each class. My possible solution is to put additional methods inside the class.
Comment